Data Structures¶
DataArray¶
xray.DataArray is xray’s implementation of a labeled, multi-dimensional array. It has several key properties:
- values: a numpy.ndarray holding the array’s values
- dims: dimension names for each axis (e.g., ('x', 'y', 'z'))
- coords: a dict-like container of arrays (coordinates) that label each point (e.g., 1-dimensional arrays of numbers, datetime objects or strings)
- attrs: an OrderedDict to hold arbitrary metadata (attributes)
xray uses dims and coords to enable its core metadata aware operations. Dimensions provide names that xray uses instead of the axis argument found in many numpy functions. Coordinates enable fast label based indexing and alignment, building on the functionality of the index found on a pandas DataFrame or Series.
DataArray objects also can have a name and can hold arbitrary metadata in the form of their attrs property (an ordered dictionary). Names and attributes are strictly for users and user-written code: xray makes no attempt to interpret them, and propagates them only in unambiguous cases (see FAQ, What is your approach to metadata?).
Creating a DataArray¶
The DataArray constructor takes a multi-dimensional array of values (e.g., a numpy ndarray), a list or dictionary of coordinates label and a list of dimension names:
In [1]: data = np.random.rand(4, 3)
In [2]: locs = ['IA', 'IL', 'IN']
In [3]: times = pd.date_range('2000-01-01', periods=4)
In [4]: foo = xray.DataArray(data, coords=[times, locs], dims=['time', 'space'])
In [5]: foo
Out[5]:
<xray.DataArray (time: 4, space: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
All of these arguments (except for data) are optional, and will be filled in with default values:
In [6]: xray.DataArray(data)
Out[6]:
<xray.DataArray (dim_0: 4, dim_1: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
* dim_0 (dim_0) int64 0 1 2 3
* dim_1 (dim_1) int64 0 1 2
As you can see, dimensions and coordinate arrays corresponding to each dimension are always present. This behavior is similar to pandas, which fills in index values in the same way.
The data array constructor also supports supplying coords as a list of (dim, ticks[, attrs]) pairs with length equal to the number of dimensions:
In [7]: xray.DataArray(data, coords=[('time', times), ('space', locs)])
Out[7]:
<xray.DataArray (time: 4, space: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
Yet another option is to supply coords in the form of a dictionary where the values are scaler values, 1D arrays or tuples (in the same form as the dataarray constructor). This form lets you supply other coordinates than those corresponding to dimensions (more on these later):
In [8]: xray.DataArray(data, coords={'time': times, 'space': locs, 'const': 42,
...: 'ranking': ('space', [1, 2, 3])},
...: dims=['time', 'space'])
...:
Out[8]:
<xray.DataArray (time: 4, space: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
ranking (space) int64 1 2 3
* space (space) |S2 'IA' 'IL' 'IN'
const int64 42
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
You can also create a DataArray by supplying a pandas Series, DataFrame or Panel, in which case any non-specified arguments in the DataArray constructor will be filled in from the pandas object:
In [9]: df = pd.DataFrame({'x': [0, 1], 'y': [2, 3]}, index=['a', 'b'])
In [10]: df.index.name = 'abc'
In [11]: df.columns.name = 'xyz'
In [12]: df
Out[12]:
xyz x y
abc
a 0 2
b 1 3
In [13]: xray.DataArray(df)
Out[13]:
<xray.DataArray (abc: 2, xyz: 2)>
array([[0, 2],
[1, 3]])
Coordinates:
* abc (abc) object 'a' 'b'
* xyz (xyz) object 'x' 'y'
xray does not (yet!) support labeling coordinate values with a pandas.MultiIndex (see GH164). However, the alternate from_series constructor will automatically unpack any hierarchical indexes it encounters by expanding the series into a multi-dimensional array, as described in Working with pandas.
DataArray properties¶
Let’s take a look at the important properties on our array:
In [14]: foo.values
Out[14]:
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
In [15]: foo.dims
Out[15]: ('time', 'space')
In [16]: foo.coords
Out[16]:
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
In [17]: foo.attrs
Out[17]: OrderedDict()
In [18]: print(foo.name)
None
You can even modify values inplace:
In [19]: foo.values = 1.0 * foo.values
Note
The array values in a DataArray have a single (homogeneous) data type. To work with heterogeneous or structured data types in xray, use coordinates, or put separate DataArray objects in a single Dataset (see below).
Now fill in some of that missing metadata:
In [20]: foo.name = 'foo'
In [21]: foo.attrs['units'] = 'meters'
In [22]: foo
Out[22]:
<xray.DataArray 'foo' (time: 4, space: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
Attributes:
units: meters
The rename() method is another option, returning a new data array:
In [23]: foo.rename('bar')
Out[23]:
<xray.DataArray 'bar' (time: 4, space: 3)>
array([[ 0.127, 0.967, 0.26 ],
[ 0.897, 0.377, 0.336],
[ 0.451, 0.84 , 0.123],
[ 0.543, 0.373, 0.448]])
Coordinates:
* space (space) |S2 'IA' 'IL' 'IN'
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
Attributes:
units: meters
DataArray Coordinates¶
The coords property is dict like. Individual coordinates can be accessed from the coordinates by name, or even by indexing the data array itself:
In [24]: foo.coords['time']
Out[24]:
<xray.DataArray 'time' (time: 4)>
array(['1999-12-31T18:00:00.000000000-0600', '2000-01-01T18:00:00.000000000-0600',
'2000-01-02T18:00:00.000000000-0600', '2000-01-03T18:00:00.000000000-0600'], dtype='datetime64[ns]')
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
In [25]: foo['time']
Out[25]:
<xray.DataArray 'time' (time: 4)>
array(['1999-12-31T18:00:00.000000000-0600', '2000-01-01T18:00:00.000000000-0600',
'2000-01-02T18:00:00.000000000-0600', '2000-01-03T18:00:00.000000000-0600'], dtype='datetime64[ns]')
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
These are also DataArray objects, which contain tick-labels for each dimension.
Coordinates can also be set or removed by using the dictionary like syntax:
In [26]: foo['ranking'] = ('space', [1, 2, 3])
In [27]: foo.coords
Out[27]:
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
ranking (space) int64 1 2 3
In [28]: del foo['ranking']
In [29]: foo.coords
Out[29]:
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
Dataset¶
xray.Dataset is xray’s multi-dimensional equivalent of a DataFrame. It is a dict-like container of labeled arrays (DataArray objects) with aligned dimensions. It is designed as an in-memory representation of the data model from the netCDF file format.
In addition to the dict-like interface of the dataset itself, which can be used to access any variable in a dataset, datasets have four key properties:
- dims: a dictionary mapping from dimension names to the fixed length of each dimension (e.g., {'x': 6, 'y': 6, 'time': 8})
- data_vars: a dict-like container of DataArrays corresponding to variables
- coords: another dict-like container of DataArrays intended to label points used in data_vars (e.g., 1-dimensional arrays of numbers, datetime objects or strings)
- attrs: an OrderedDict to hold arbitrary metadata
The distinction between whether a variables falls in data or coordinates (borrowed from CF conventions) is mostly semantic, and you can probably get away with ignoring it if you like: dictionary like access on a dataset will supply variables found in either category. However, xray does make use of the distinction for indexing and computations. Coordinates indicate constant/fixed/independent quantities, unlike the varying/measured/dependent quantities that belong in data.
Here is an example of how we might structure a dataset for a weather forecast:
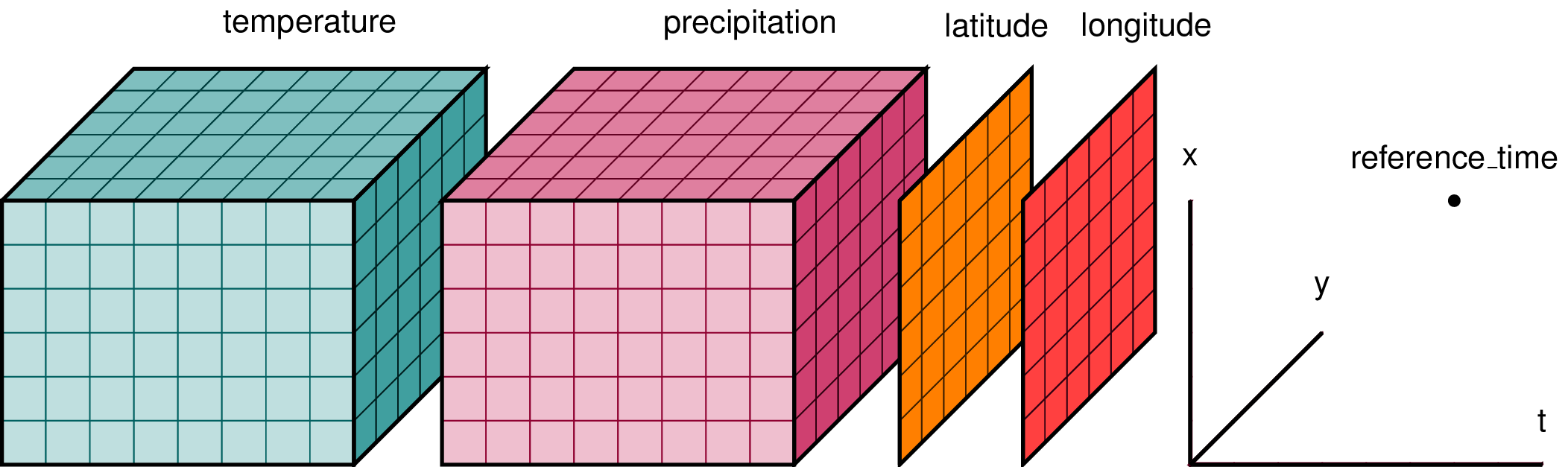
In this example, it would be natural to call temperature and precipitation “data variables” and all the other arrays “coordinate variables” because they label the points along the dimensions. (see [1] for more background on this example).
Creating a Dataset¶
To make an Dataset from scratch, supply dictionaries for any variables, coordinates and attributes you would like to insert into the dataset.
For the vars and coords arguments, keys should be the name of the variable and values should be scalars, 1d arrays or tuples of the form (dims, data[, attrs]) sufficient to label each array:
- dims should be a sequence of strings.
- data should be a numpy.ndarray (or array-like object) that has a dimensionality equal to the length of dims.
- attrs is an arbitrary Python dictionary for storing metadata associated with a particular array.
Let’s create some fake data for the example we show above:
In [30]: temp = 15 + 8 * np.random.randn(2, 2, 3)
In [31]: precip = 10 * np.random.rand(2, 2, 3)
In [32]: lon = [[-99.83, -99.32], [-99.79, -99.23]]
In [33]: lat = [[42.25, 42.21], [42.63, 42.59]]
# for real use cases, its good practice to supply array attributes such as
# units, but we won't bother here for the sake of brevity
In [34]: ds = xray.Dataset({'temperature': (['x', 'y', 'time'], temp),
....: 'precipitation': (['x', 'y', 'time'], precip)},
....: coords={'lon': (['x', 'y'], lon),
....: 'lat': (['x', 'y'], lat),
....: 'time': pd.date_range('2014-09-06', periods=3),
....: 'reference_time': pd.Timestamp('2014-09-05')})
....:
In [35]: ds
Out[35]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
lat (x, y) float64 42.25 42.21 42.63 42.59
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
Data variables:
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
Notice that we did not explicitly include coordinates for the “x” or “y” dimensions, so they were filled in array of ascending integers of the proper length.
We can also pass xray.DataArray objects as values in the dictionary instead of tuples:
In [36]: xray.Dataset({'bar': foo})
Out[36]:
<xray.Dataset>
Dimensions: (space: 3, time: 4)
Coordinates:
* time (time) datetime64[ns] 2000-01-01 2000-01-02 2000-01-03 2000-01-04
* space (space) |S2 'IA' 'IL' 'IN'
Data variables:
bar (time, space) float64 0.127 0.9667 0.2605 0.8972 0.3767 0.3362 ...
You can also create an dataset from a pandas.DataFrame with Dataset.from_dataframe or from a netCDF file on disk with open_dataset(). See Working with pandas and Serialization and IO.
Dataset contents¶
Dataset implements the Python dictionary interface, with values given by xray.DataArray objects:
In [37]: 'temperature' in ds
Out[37]: True
In [38]: ds.keys()
Out[38]:
['precipitation',
'temperature',
'lat',
'reference_time',
'lon',
'time',
'x',
'y']
In [39]: ds['temperature']
Out[39]:
<xray.DataArray 'temperature' (x: 2, y: 2, time: 3)>
array([[[ 11.041, 23.574, 20.772],
[ 9.346, 6.683, 17.175]],
[[ 11.6 , 19.536, 17.21 ],
[ 6.301, 9.61 , 15.909]]])
Coordinates:
* y (y) int64 0 1
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
lat (x, y) float64 42.25 42.21 42.63 42.59
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
The valid keys include each listed coordinate and data variable.
Data and coordinate variables are also contained separately in the data_vars and coords dictionary-like attributes:
In [40]: ds.data_vars
Out[40]:
Data variables:
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 0.3777 ...
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
In [41]: ds.coords
Out[41]:
Coordinates:
lat (x, y) float64 42.25 42.21 42.63 42.59
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
Finally, like data arrays, datasets also store arbitrary metadata in the form of attributes:
In [42]: ds.attrs
Out[42]: OrderedDict()
In [43]: ds.attrs['title'] = 'example attribute'
In [44]: ds
Out[44]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
lat (x, y) float64 42.25 42.21 42.63 42.59
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
Data variables:
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
Attributes:
title: example attribute
xray does not enforce any restrictions on attributes, but serialization to some file formats may fail if you use objects that are not strings, numbers or numpy.ndarray objects.
As a useful shortcut, you can use attribute style access for reading (but not setting) variables and attributes:
In [45]: ds.temperature
Out[45]:
<xray.DataArray 'temperature' (x: 2, y: 2, time: 3)>
array([[[ 11.041, 23.574, 20.772],
[ 9.346, 6.683, 17.175]],
[[ 11.6 , 19.536, 17.21 ],
[ 6.301, 9.61 , 15.909]]])
Coordinates:
* y (y) int64 0 1
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
lat (x, y) float64 42.25 42.21 42.63 42.59
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
This is particularly useful in an exploratory context, because you can tab-complete these variable names with tools like IPython.
Dictionary like methods¶
We can update a dataset in-place using Python’s standard dictionary syntax. For example, to create this example dataset from scratch, we could have written:
In [46]: ds = xray.Dataset()
In [47]: ds['temperature'] = (('x', 'y', 'time'), temp)
In [48]: ds['precipitation'] = (('x', 'y', 'time'), precip)
In [49]: ds.coords['lat'] = (('x', 'y'), lat)
In [50]: ds.coords['lon'] = (('x', 'y'), lon)
In [51]: ds.coords['time'] = pd.date_range('2014-09-06', periods=3)
In [52]: ds.coords['reference_time'] = pd.Timestamp('2014-09-05')
To change the variables in a Dataset, you can use all the standard dictionary methods, including values, items, __delitem__, get and update(). Note that assigning a DataArray object to a Dataset variable using __setitem__ or update will automatically align the array(s) to the original dataset’s indexes.
Modifying datasets¶
You can copy a Dataset by using the copy() method:
In [53]: ds.copy()
Out[53]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
Data variables:
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
By default, the copy is shallow, so only the container will be copied: the arrays in the Dataset will still be stored in the same underlying numpy.ndarray objects. You can copy all data by supplying the argument deep=True.
You also can select and drop an explicit list of variables by using the by indexing with a list of names or using the drop() methods to return a new Dataset. These operations keep around coordinates:
In [54]: ds[['temperature']]
Out[54]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
lat (x, y) float64 42.25 42.21 42.63 42.59
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* y (y) int64 0 1
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
Data variables:
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
In [55]: ds[['x']]
Out[55]:
<xray.Dataset>
Dimensions: (x: 2)
Coordinates:
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
Data variables:
*empty*
If a dimension name is given as an argument to drop, it also drops all variables that use that dimension:
In [56]: ds.drop('time')
Out[56]:
<xray.Dataset>
Dimensions: (x: 2, y: 2)
Coordinates:
* x (x) int64 0 1
* y (y) int64 0 1
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
Data variables:
*empty*
Another useful option is the ability to rename the variables in a dataset:
In [57]: ds.rename({'temperature': 'temp', 'precipitation': 'precip'})
Out[57]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
Data variables:
temp (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
precip (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
Finally, you can use swap_dims() to swap dimension and non-dimension variables:
In [58]: ds.coords['day'] = ('time', [6, 7, 8])
In [59]: ds.swap_dims({'time': 'day'})
Out[59]:
<xray.Dataset>
Dimensions: (day: 3, x: 2, y: 2)
Coordinates:
time (day) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
* day (day) int64 6 7 8
Data variables:
temperature (x, y, day) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
precipitation (x, y, day) float64 5.904 2.453 3.404 9.847 9.195 0.3777 ...
Coordinates¶
Coordinates are ancillary variables stored for DataArray and Dataset objects in the coords attribute:
In [60]: ds.coords
Out[60]:
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
day (time) int64 6 7 8
Unlike attributes, xray does interpret and persist coordinates in operations that transform xray objects.
One dimensional coordinates with a name equal to their sole dimension (marked by * when printing a dataset or data array) take on a special meaning in xray. They are used for label based indexing and alignment, like the index found on a pandas DataFrame or Series. Indeed, these “dimension” coordinates use a pandas.Index internally to store their values.
Other than for indexing, xray does not make any direct use of the values associated with coordinates. Coordinates with names not matching a dimension are not used for alignment or indexing, nor are they required to match when doing arithmetic (see Coordinates).
Modifying coordinates¶
To entirely add or removing coordinate arrays, you can use dictionary like syntax, as shown above.
To convert back and forth between data and coordinates, you can use the set_coords() and reset_coords() methods:
In [61]: ds.reset_coords()
Out[61]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
Data variables:
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
day (time) int64 6 7 8
In [62]: ds.set_coords(['temperature', 'precipitation'])
Out[62]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* x (x) int64 0 1
* y (y) int64 0 1
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
lat (x, y) float64 42.25 42.21 42.63 42.59
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
reference_time datetime64[ns] 2014-09-05
day (time) int64 6 7 8
Data variables:
*empty*
In [63]: ds['temperature'].reset_coords(drop=True)
Out[63]:
<xray.DataArray 'temperature' (x: 2, y: 2, time: 3)>
array([[[ 11.041, 23.574, 20.772],
[ 9.346, 6.683, 17.175]],
[[ 11.6 , 19.536, 17.21 ],
[ 6.301, 9.61 , 15.909]]])
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* y (y) int64 0 1
* x (x) int64 0 1
Notice that these operations skip coordinates with names given by dimensions, as used for indexing. This mostly because we are not entirely sure how to design the interface around the fact that xray cannot store a coordinate and variable with the name but different values in the same dictionary. But we do recognize that supporting something like this would be useful.
Coordinates methods¶
Coordinates objects also have a few useful methods, mostly for converting them into dataset objects:
In [64]: ds.coords.to_dataset()
Out[64]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
lat (x, y) float64 42.25 42.21 42.63 42.59
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* y (y) int64 0 1
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
day (time) int64 6 7 8
Data variables:
*empty*
The merge method is particularly interesting, because it implements the same logic used for merging coordinates in arithmetic operations (see Computation):
In [65]: alt = xray.Dataset(coords={'z': [10], 'lat': 0, 'lon': 0})
In [66]: ds.coords.merge(alt.coords)
Out[66]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2, z: 1)
Coordinates:
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
* y (y) int64 0 1
* x (x) int64 0 1
reference_time datetime64[ns] 2014-09-05
day (time) int64 6 7 8
* z (z) int64 10
Data variables:
*empty*
The coords.merge method may be useful if you want to implement your own binary operations that act on xray objects. In the future, we hope to write more helper functions so that you can easily make your functions act like xray’s built-in arithmetic.
Indexes¶
To convert a coordinate (or any DataArray) into an actual pandas.Index, use the to_index() method:
In [67]: ds['time'].to_index()
Out[67]: DatetimeIndex(['2014-09-06', '2014-09-07', '2014-09-08'], dtype='datetime64[ns]', name=u'time', freq='D', tz=None)
A useful shortcut is the indexes property (on both DataArray and Dataset), which lazily constructs a dictionary whose keys are given by each dimension and whose the values are Index objects:
In [68]: ds.indexes
Out[68]:
time: DatetimeIndex(['2014-09-06', '2014-09-07', '2014-09-08'], dtype='datetime64[ns]', name=u'time', freq='D', tz=None)
x: Int64Index([0, 1], dtype='int64', name=u'x')
y: Int64Index([0, 1], dtype='int64', name=u'y')
Converting datasets and arrays¶
To convert from a Dataset to a DataArray, use to_array():
In [69]: arr = ds.to_array()
In [70]: arr
Out[70]:
<xray.DataArray (variable: 2, x: 2, y: 2, time: 3)>
array([[[[ 11.041, 23.574, 20.772],
[ 9.346, 6.683, 17.175]],
[[ 11.6 , 19.536, 17.21 ],
[ 6.301, 9.61 , 15.909]]],
[[[ 5.904, 2.453, 3.404],
[ 9.847, 9.195, 0.378]],
[[ 8.615, 7.536, 4.052],
[ 3.435, 1.709, 3.947]]]])
Coordinates:
reference_time datetime64[ns] 2014-09-05
lat (x, y) float64 42.25 42.21 42.63 42.59
* y (y) int64 0 1
* x (x) int64 0 1
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
* variable (variable) |S13 'temperature' 'precipitation'
day (time) int64 6 7 8
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
This method broadcasts all data variables in the dataset against each other, then concatenates them along a new dimension into a new array while preserving coordinates.
To convert back from a DataArray to a Dataset, use to_dataset():
In [71]: arr.to_dataset(dim='variable')
Out[71]:
<xray.Dataset>
Dimensions: (time: 3, x: 2, y: 2)
Coordinates:
reference_time datetime64[ns] 2014-09-05
lat (x, y) float64 42.25 42.21 42.63 42.59
* y (y) int64 0 1
* x (x) int64 0 1
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
day (time) int64 6 7 8
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
Data variables:
temperature (x, y, time) float64 11.04 23.57 20.77 9.346 6.683 17.17 ...
precipitation (x, y, time) float64 5.904 2.453 3.404 9.847 9.195 ...
The broadcasting behavior of to_array means that the resulting array includes the union of data variable dimensions:
In [72]: ds2 = xray.Dataset({'a': 0, 'b': ('x', [3, 4, 5])})
# the input dataset has 4 elements
In [73]: ds2
Out[73]:
<xray.Dataset>
Dimensions: (x: 3)
Coordinates:
* x (x) int64 0 1 2
Data variables:
a int64 0
b (x) int64 3 4 5
# the resulting array has 6 elements
In [74]: ds2.to_array()
Out[74]:
<xray.DataArray (variable: 2, x: 3)>
array([[0, 0, 0],
[3, 4, 5]])
Coordinates:
* variable (variable) |S1 'a' 'b'
* x (x) int64 0 1 2
Otherwise, the result could not be represented as an orthogonal array.
If you use to_dataset without supplying the dim argument, the DataArray will be converted into a Dataset of one variable:
In [75]: arr.to_dataset(name='combined')
Out[75]:
<xray.Dataset>
Dimensions: (time: 3, variable: 2, x: 2, y: 2)
Coordinates:
reference_time datetime64[ns] 2014-09-05
lon (x, y) float64 -99.83 -99.32 -99.79 -99.23
* y (y) int64 0 1
* variable (variable) |S13 'temperature' 'precipitation'
* time (time) datetime64[ns] 2014-09-06 2014-09-07 2014-09-08
lat (x, y) float64 42.25 42.21 42.63 42.59
* x (x) int64 0 1
day (time) int64 6 7 8
Data variables:
combined (variable, x, y, time) float64 11.04 23.57 20.77 9.346 ...
[1] | Latitude and longitude are 2D arrays because the dataset uses projected coordinates. reference_time refers to the reference time at which the forecast was made, rather than time which is the valid time for which the forecast applies. |